This blog entry is part of the series of blogs to productionise a docker image in Kubernetes for enterprises. In our previous article, we saw a quick way of packaging and deploying Docker images to Kubernetes using Helm. In this entry, we will see a way of templating in Helm to modify ports at deploy time.
Minikube is a tool that runs a single-node Kubernetes cluster in a virtual machine on your personal computer. Deploying it in Minikube normally means you will be able to deploy your Docker images in most other versions of Kubernetes without many modifications.
For the sake of simplicity, we shall pick a couple of docker images from open libraries.
- Mariadb is a community-developed fork of MySQL DB intended to remain free under the GNU GPL
- PhpMyAdmin A web interface for MySQL and MariaDB administration.
This is a quick way of a simulating an application stack with a 2-tier based web architecture and orchestration of multiple docker containers.
Helm is arguably the most famous package manager for Kubernetes resources. The closest analogies for Helm are Maven in Java world, Yum, Brew and apt-get. All of us must have used at least one of them and whoever has experienced a package manager will appreciate the ease of shareability and lifecycle management of the packages.
For Helm, there are three important concepts:
- The chart is a bundle of information necessary to create an instance of a Kubernetes application.
- The config contains configuration information that can be merged into a packaged chart to create a releasable object.
- A release is a running instance of a chart, combined with a specific config.
Templates generate manifest files, which are YAML-formatted resource descriptions that Kubernetes can understand.
There are loads of publicly available charts for MariaDB and Phpmyadmin but for the sake of this article, we will use the helm charts(Git Path) we created in the article as a baseline.
Start Minikube
Start a quick single-node cluster
minikube start
Login to Docker
docker login
Install Helm by following the instructions here.
Templating MariaDB
Update mariadb/templates/service.yaml to replace hardcoded value of 3306 with a template variable “port”. More details on templating
apiVersion: v1 kind: Service metadata: labels: app: mariadb name: mariadb spec: ports: - name: tcp-{{ .Values.port }} port: {{ .Values.port }} targetPort: {{ .Values.port }} selector: app: mariadb
Create mariadb/values.yaml
port: 3306
Lint the chart to examine the chart for possible issues
helm lint mariadb/ ==> Linting mariadb [INFO] Chart.yaml: icon is recommended 1 chart(s) linted, 0 chart(s) failed
Package the chart to examine the chart for possible issues
helm package mariadb/ Successfully packaged chart and saved it to: /Users/thiru/git/aicloudpods/tutorials/helm/basic-1/mariadb-0.1.0.tgz
Templating PhpMyAdmin
Update phpmyadmin/templates/service.yaml to replace hardcoded value of ports with a template variables. More details on templating
apiVersion: v1 kind: Service metadata: labels: app: phpmyadmin name: phpmyadmin spec: ports: - name: http-{{ .Values.port.target }} port: {{ .Values.port.source }} targetPort: {{ .Values.port.target }} selector: app: phpmyadmin type: LoadBalancer
Create phpmyadmin/values.yaml
port: source: 80 target: 8080
Lint the chart to examine the chart for possible issues
helm lint phpmyadmin/ ==> Linting phpmyadmin [INFO] Chart.yaml: icon is recommended 1 chart(s) linted, 0 chart(s) failed
Package the chart to examine the chart for possible issues
helm package phpmyadmin/ Successfully packaged chart and saved it to: /Users/thiru/git/aicloudpods/tutorials/helm/basic-1/phpmyadmin-0.1.0.tgz
Deploy Mariadb
Create a secret for storing the password for MariaDB root user.
kubectl create secret generic mariadb-secret --from-literal=root_pwd=changeit
Let us do a dry-run to simulate an install against the cluster which will also do a basic semantic check with name conflicts
helm install mariadb mariadb-0.1.0.tgz --dry-run --debug
After sanity checking above, we could perform an install.
helm install mariadb mariadb-0.1.0.tgz NAME: mariadb LAST DEPLOYED: Sun May 24 16:14:39 2020 NAMESPACE: default STATUS: deployed REVISION: 1 TEST SUITE: None
Deploy PhpMyAdmin with source port overridden to 8090
Let us do a dry-run to simulate an install against the cluster which will also do a basic semantic check with name conflicts
helm install phpmyadmin phpmyadmin-0.1.0.tgz --dry-run --debug --set port.source=8090
After sanity checking above, we could perform an install.
helm install phpmyadmin phpmyadmin-0.1.0.tgz --set port.source=8090 NAME: phpmyadmin LAST DEPLOYED: Sun May 24 16:14:55 2020 NAMESPACE: default STATUS: deployed REVISION: 1 TEST SUITE: None
Get the list of helm releases installed
helm list NAME NAMESPACE REVISION UPDATED STATUS CHART APP VERSION mariadb default 1 2020-05-24 16:14:39.240828 +0100 BST deployed mariadb-0.1.0 1.16.0 phpmyadmin default 1 2020-05-24 16:14:55.431947 +0100 BST deployed phpmyadmin-0.1.0 1.16.0
Get the list of running deployments, pods, services and configmaps
kubectl get deployments,pods,svc,configmaps,secrets NAME READY UP-TO-DATE AVAILABLE AGE deployment.apps/mariadb 1/1 1 1 29m deployment.apps/phpmyadmin 1/1 1 1 12s NAME READY STATUS RESTARTS AGE pod/mariadb-b5b4685bf-w2dtv 1/1 Running 0 29m pod/phpmyadmin-b6f5cbfc6-sjm5d 1/1 Running 0 12s NAME TYPE CLUSTER-IP EXTERNAL-IP PORT(S) AGE service/kubernetes ClusterIP 10.96.0.1 443/TCP 10d service/mariadb ClusterIP 10.101.38.216 3306/TCP 29m service/phpmyadmin LoadBalancer 10.96.186.180 8090:31024/TCP 12s NAME DATA AGE configmap/phpmyadmin-config 1 12s NAME TYPE DATA AGE secret/default-token-9bp76 kubernetes.io/service-account-token 3 10d secret/mariadb-secret Opaque 1 29m secret/sh.helm.release.v1.mariadb.v1 helm.sh/release.v1 1 29m secret/sh.helm.release.v1.phpmyadmin.v1 helm.sh/release.v1 1 12s
View the phpmyadmin service in browser using the below command
minikube service phpmyadmin
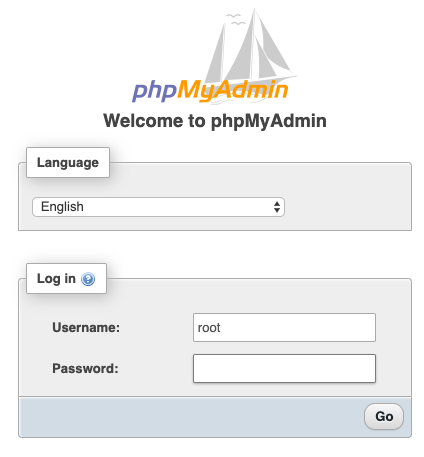
Login using ‘root’ as Username and ‘changeit’ as password. Click on the SQL tab and execute a sample query “select 1 from dual”, hit “Go” button to see results(as below). Also, this will prove end to end connectivity.
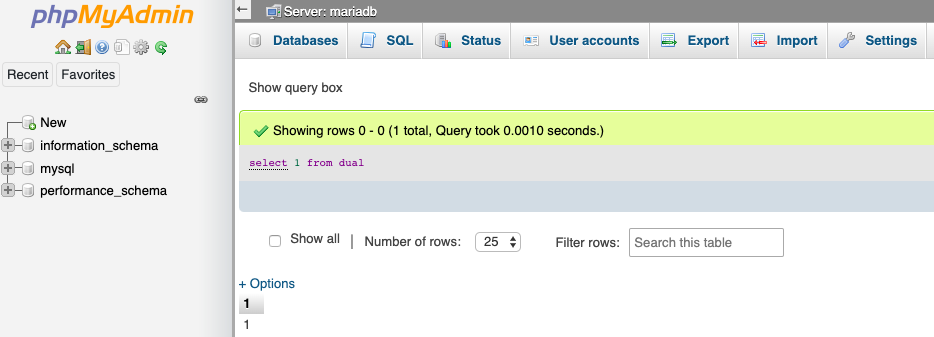
Delete the resources
helm uninstall mariadb helm uninstall phpmyadmin kubectl delete secret mariadb-secret
This is a quick demonstration of templating in Helm charts and all source code used in this article are available in https://github.com/aicloudpods/tutorials/tree/master/helm/templating. Helm offers a lot more features like templating, dependency management, etc., and we will explore each of them in future articles. In the next article, we will explore ChartMuseum, a centralised repository for sharing charts within and outside your organisation.